mirror of
https://git.jami.net/savoirfairelinux/jami-client-qt.git
synced 2025-07-15 21:15:24 +02:00
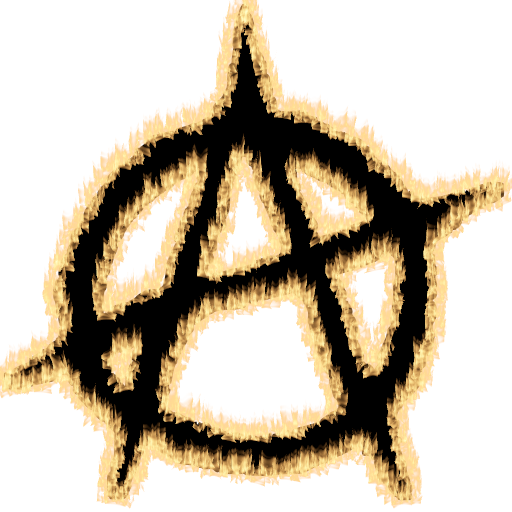
This commit adds a basic crash-report system that can be optionally configured to automatically send minidump crash-reports in addition to product versions and a platform description including the OS name and CPU architecture. Reports can be received at a configured REST endpoint(POST). This endpoint URL can be configured using a CMake variable `CRASH_REPORT_URL` which defaults to "http://localhost:8080/submit". - Introduces a new CMake option `ENABLE_CRASHREPORTS`, defaulting to OFF. This allows developers to enable crash reporting features at build time selectively. We also define a new macro with the same name to expose the state to QML in order to hide the UI components if needed. - Implemented conditional inclusion of crashpad dependencies using `ENABLE_CRASHREPORTS`. If set, `ENABLE_CRASHPAD` is also enabled (other crash reporters exist and we may want to use them). - 2 new application settings are added: `EnableCrashReporting` and `EnableAutomaticCrashReporting`. Default settings make it so crash-reports are generated but not automatically sent. With this default configuration, users will be prompted upon application start to confirm the report upload. Additionally, users may opt-in in order to have reports sent automatically at crash-time. Gitlab: #1454 Change-Id: I53edab2dae210240a99272479381695fce1e221b
51 lines
No EOL
1.8 KiB
Python
51 lines
No EOL
1.8 KiB
Python
#!/usr/bin/env python3
|
|
|
|
import os
|
|
from flask import Flask, request
|
|
import json
|
|
|
|
app = Flask(__name__)
|
|
|
|
@app.route('/submit', methods=['POST'])
|
|
def submit():
|
|
try:
|
|
print("Received a crash report GUID: %s" % request.form.get('guid', 'No GUID provided'))
|
|
file_storage = request.files.get('upload_file_minidump')
|
|
dump_id = ""
|
|
if file_storage:
|
|
dump_id = file_storage.filename
|
|
|
|
# Create a directory to store the crash reports if it doesn't exist
|
|
base_path = 'crash_reports'
|
|
if not os.path.exists(base_path):
|
|
os.makedirs(base_path)
|
|
|
|
filepath = os.path.join(base_path, dump_id)
|
|
|
|
# Attempt to write the file, fail gracefully if it already exists
|
|
if os.path.exists(filepath):
|
|
print(f"File {filepath} already exists.")
|
|
return 'File already exists', 409
|
|
with open(filepath, 'wb') as f:
|
|
f.write(file_storage.read())
|
|
print(f"File saved successfully at {filepath}")
|
|
|
|
# Now save the metadata in {request.form} as separate filename <UID>.info.
|
|
# We assume the data is a JSON string.
|
|
metadata_filepath = os.path.join(base_path, f"{dump_id}.info")
|
|
with open(metadata_filepath, 'w') as f:
|
|
f.write(str(json.dumps(dict(request.form), indent=4)))
|
|
else:
|
|
print("No file found for the key 'upload_file_minidump'")
|
|
return 'No file found', 400
|
|
|
|
return 'Crash report received', 200
|
|
except OSError as e:
|
|
print(f"Error creating directory or writing file: {e}")
|
|
return 'Internal Server Error', 500
|
|
except Exception as e:
|
|
print(f"An unexpected error occurred: {e}")
|
|
return 'Internal Server Error', 500
|
|
|
|
if __name__ == '__main__':
|
|
app.run(port=8080, debug=True) |