mirror of
https://git.jami.net/savoirfairelinux/jami-client-qt.git
synced 2025-07-03 23:25:28 +02:00
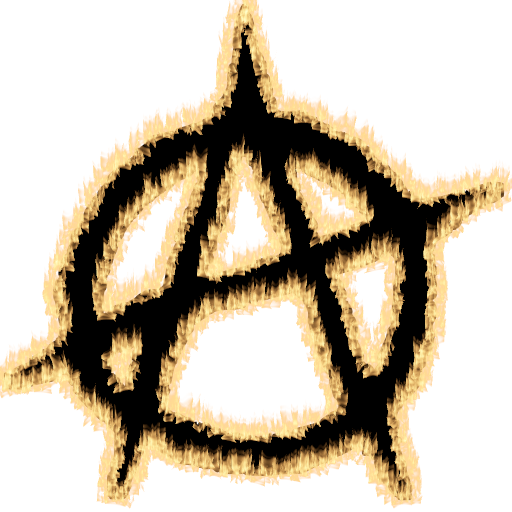
Using a custom getter setter cause the setting of the value to require manual changed signal emission and in addition to being error prone, this prevents the changed signal from being emitted upon initial selection. Some excess workaround code may be removed in following commits. This patch also refactors the banned contact signaling as part of an accountadapter connection cleanup. Change-Id: I73fb751001e53e086adc7a6a8d706671c2878a77
94 lines
No EOL
3.1 KiB
C++
94 lines
No EOL
3.1 KiB
C++
/*
|
|
* Copyright (C) 2021 by Savoir-faire Linux
|
|
* Author: Albert Babí Oller <albert.babi@savoirfairelinux.com>
|
|
* Author: Mingrui Zhang <mingrui.zhang@savoirfairelinux.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 3 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <https://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include "globaltestenvironment.h"
|
|
|
|
TestEnvironment globalEnv;
|
|
|
|
/*!
|
|
* Test fixture for AccountAdapter testing
|
|
*/
|
|
class AccountFixture : public ::testing::Test
|
|
{
|
|
public:
|
|
// Prepare unit test context. Called at
|
|
// prior each unit test execution
|
|
void SetUp() override {}
|
|
|
|
// Close unit test context. Called
|
|
// after each unit test ending
|
|
void TearDown() override {}
|
|
};
|
|
|
|
/*!
|
|
* WHEN There is no account initially.
|
|
* THEN Account list should be empty.
|
|
*/
|
|
TEST_F(AccountFixture, InitialAccountListCheck)
|
|
{
|
|
auto accountListSize = globalEnv.lrcInstance->accountModel().getAccountList().size();
|
|
|
|
ASSERT_EQ(accountListSize, 0);
|
|
}
|
|
|
|
/*!
|
|
* WHEN An SIP account is created.
|
|
* THEN The size of the account list should be one.
|
|
*/
|
|
TEST_F(AccountFixture, CreateSIPAccountTest)
|
|
{
|
|
// AccountAdded signal spy
|
|
QSignalSpy accountAddedSpy(&globalEnv.lrcInstance->accountModel(),
|
|
&NewAccountModel::accountAdded);
|
|
|
|
// Create SIP Acc
|
|
globalEnv.accountAdapter->createSIPAccount(QVariantMap());
|
|
|
|
QVERIFY(accountAddedSpy.wait());
|
|
QCOMPARE(accountAddedSpy.count(), 1);
|
|
|
|
QList<QVariant> accountAddedArguments = accountAddedSpy.takeFirst();
|
|
QVERIFY(accountAddedArguments.at(0).type() == QVariant::String);
|
|
|
|
// Select the created account
|
|
globalEnv.lrcInstance->set_currentAccountId(accountAddedArguments.at(0).toString());
|
|
|
|
auto accountListSize = globalEnv.lrcInstance->accountModel().getAccountList().size();
|
|
ASSERT_EQ(accountListSize, 1);
|
|
|
|
// Make sure the account setup is done
|
|
QSignalSpy accountStatusChangedSpy(&globalEnv.lrcInstance->accountModel(),
|
|
&NewAccountModel::accountStatusChanged);
|
|
|
|
QVERIFY(accountStatusChangedSpy.wait());
|
|
QCOMPARE(accountStatusChangedSpy.count(), 1);
|
|
|
|
// Remove the account
|
|
globalEnv.lrcInstance->accountModel().removeAccount(
|
|
globalEnv.lrcInstance->get_currentAccountId());
|
|
|
|
QSignalSpy accountRemovedSpy(&globalEnv.lrcInstance->accountModel(),
|
|
&NewAccountModel::accountRemoved);
|
|
|
|
QVERIFY(accountRemovedSpy.wait());
|
|
QCOMPARE(accountRemovedSpy.count(), 1);
|
|
|
|
accountListSize = globalEnv.lrcInstance->accountModel().getAccountList().size();
|
|
ASSERT_EQ(accountListSize, 0);
|
|
} |